January 8th, 2025
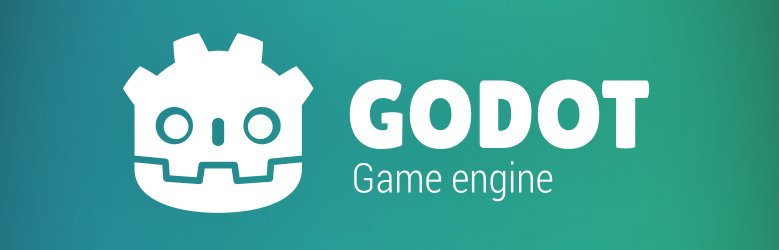
We're happy to announce the release of our spine-godot GDExtension! It's a major step forward for integrating Spine animations into Godot projects. This release brings all the power of Spine's animation system to Godot without requiring custom engine builds, making it easier than ever for developers to harness Spine's capabilities. It also makes it easier to target consoles down the road. Get in touch with W4Games if you require console support for your Godot game.
The spine-godot GDExtension supports all Spine features. However, a GDExtension has less integration with the Godot editor, so a few editor features are missing:
- Support for
AnimationPlayer
via SpineAnimationTrackNode
. This would require Godot to expose the AnimationPlayer
API in GDExtension. - Dedicated C# bindings. See this Godot proposal on the state of C# support for GDExtensions.
- Support for editor selection rects. See this Godot proposal for more information.
To start using the spine-godot GDExtension, visit the official documentation. The docs include detailed setup instructions, supported features, and example projects to help you hit the ground running.
We're excited to see what you'll create with Spine and Godot!
Discuss this blog post on the Spine forum.
December 6th, 2024

We've upgraded our Spine runtime for three.js!
Three.js is a library for creating animated 3D graphics in the browser. While it's not always the first choice for Spine users, it's an excellent option if you want to combine 2D and 3D elements. Our runtime allows you to bring your 2D Spine animations into the 3D world of three.js with ease.
This upgrade aims to integrate Spine skeletons more naturally in three.js. You can now choose the material used to create your Spine objects. This means your skeletons can react to lights, casting and receiving shadows if you use materials like MeshStandardMaterial
. Alternatively, if you prefer to display your animations as they appear in the Spine editor, you can use materials like MeshBasicMaterial
that don't react to lighting.
As an example, check out this interactive demo of a raptor skeleton casting and receiving shadows:
We've added support for the "tint black" feature, ensuring spine-threejs
fully supports all Spine features.
In r160, three.js dropped support for IIFE bundles in favor of modern ECMAScript Modules (ESM). To make your life easier, we have started shipping ESM modules as well. From now on, you can use the import/export syntax in your code like this:
html
<
script type=
"importmap">
{
"imports": {
"three":
"https://cdn.jsdelivr.net/npm/three@0.171.0/build/three.module.js",
"spine-threejs":
"https://unpkg.com/@esotericsoftware/spine-threejs@4.2.67/dist/esm/spine-threejs.min.mjs" }
}
</
script>
<
script type=
"module">
import *
as THREE from "three";
import *
as spine from "spine-threejs";
...
</
script>
We upgraded the minimum supported version of three.js from r141 to r162 due to the removal of APIs that spine-threejs
relied on. Despite this change, we maintained the spine-threejs
API without introducing breaking changes. While upgrading a peer dependency is often considered a breaking change, this update was necessary to avoid locking spine-threejs
to a three.js release over a year old. We've thoroughly tested the updated spine-threejs
runtime with three.js versions up to the latest r171, and everything works as expected.
As usual, if you need assistance in using it, you can open a forum thread. If you find a bug or want to improve the runtime, feel free to open an issue or a PR on our GitHub.
Discuss this blog post on the forums!
November 7th, 2024

We've upgraded our Spine runtime for PixiJS to version 8!
PixiJS has been one of the most widely used WebGL-based rendering libraries for many years, powering countless web games and applications with its fast and flexible renderer. It is a top choice for Spine users, particularly for HTML5 game developers.
Our first official PixiJS runtime was for PixiJS v7. We want the runtime to be official so we can better support users and ensure it gets the latest features.
Meanwhile, the PixiJS team have developed and maintained their own pixi-spine
runtime for nearly 10 years. Having two separate runtimes isn't ideal, so for PixiJS v8 we collaborated with Mat Groves — the creator of PixiJS — and the PixiJS team. The result is a single spine-pixi-v8
runtime that is officially supported by us and with the PixiJS team available as needed.
Joining forces like this ensures a great Spine experience for PixiJS users! We are committed to providing timely updates and bug fixes in sync with Spine Editor releases. For Mat and the PixiJS team, this collaboration helps reduce their workload, allowing them to focus more on PixiJS itself.
With the power of PixiJS, spine-pixi-v8
becomes the first spine-ts runtime to leverage hardware acceleration through WebGPU! Here’s a minimal example that uses the WebGPU renderer, when available:
spine-pixi-v7
and spine-pixi-v8
share almost identical interfaces, allowing you to use the same documentation for both. Check out our spine-pixi documentation and explore the examples code. We've ported all PixiJS v7 examples, where you’ll see that the fields and methods remain the same.
As usual, if you need assistance in using it, you can open a forum thread. If you find a bug or want to improve the runtime, feel free to open an issue or a PR on our GitHub.
Discuss this blog post on the forums!
September 16th, 2024
We're happy to announce the general availability of our brand new spine-android
runtime.
Our new runtime makes it trivial to integrate Spine animations with your Android app, whether you are using Java or Kotlin. spine-android
is built on top of spine-libgdx, our reference runtime.
The core Spine Runtimes API is exposed as idiomatic Java, just like in our spine-libgdx runtime. On top of the core API, we've created Android-specific classes, like SpineView
. Integration with Jetpack Compose is also trivial.
spine-android
is regularly released to Maven Central and can be easily added as a dependency via Gradle.
To learn more, check out our spine-android documentation and have a look at the example projects.
Discuss this blog post on the forums!